Integrate Lit Within a Telegram Mini App
Learn how to use Lit within a Telegram Mini App.
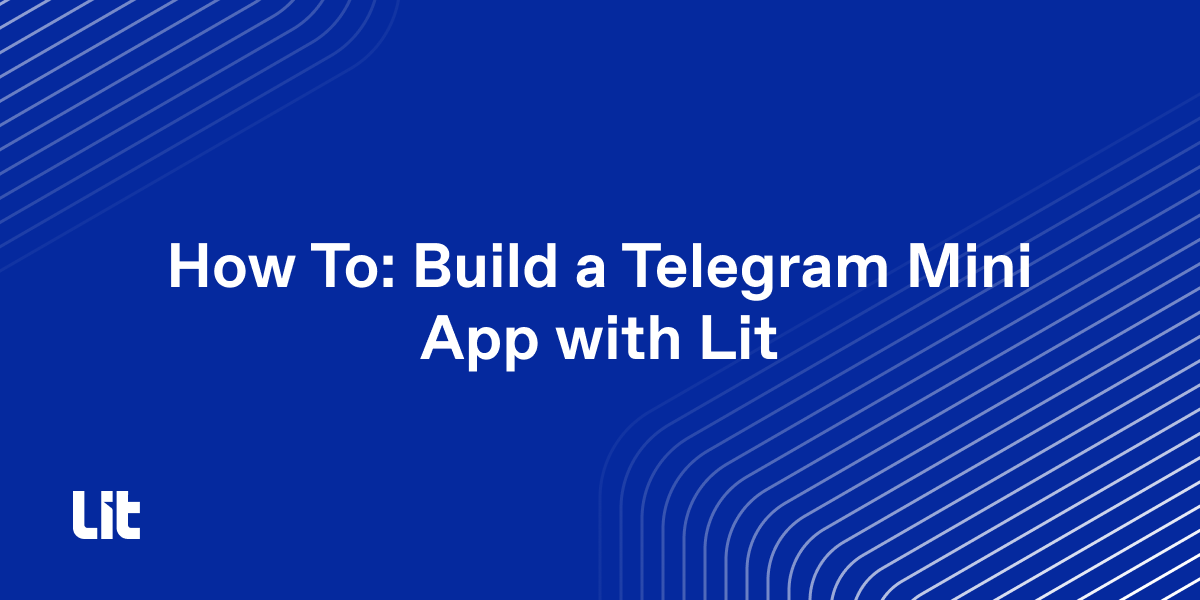
Overview
TL;DR: This guide demonstrates how to integrate Lit Protocol with Telegram Mini Apps, leveraging Programmable Key Pairs (PKPs) and custom authentication to enhance decentralized security for users in applications like trading bots. The complete codebase for this example can be found here.
Key Concepts
Lit Terminology
- Programmable Key Pairs (PKPs) are web3 wallets that can be programmed using Lit Actions
- Lit Actions are immutable JavaScript programs that run on a decentralized Lit network
- Session Signatures are used to authenticate with the Lit nodes and create a secure connection to the Lit network. They are used to request specific abilities on the Lit network, such as allowing a PKP to sign data
Telegram Mini Apps
Telegram Mini Apps are small web-based applications integrated into the Telegram platform. They enable users to access services without leaving Telegram. Currently, most developers on the Telegram App Center seem to be focused on developing Mini Apps that incorporate cryptocurrency and games, or cryptocurrency wallets for the TON blockchain.
Of course, there are other uses as well. AI chatbots, VPNs, and fantasy football are some notable mentions. Since these Mini Apps are essentially lightweight HTML5 webpages, the sky is the limit. There is a lot of potential for Web3 wallets with specialized applications, especially for any Ethereum blockchains which Telegram doesn't natively support.
How to Leverage Lit Protocol
Lit Protocol can be used to create Telegram Mini Apps that integrate advanced Web3 functionality and provide enhanced security for users. Lit can allow interaction with the Ethereum ecosystem through Telegram, allowing users to perform on-chain actions from their Telegram app.
In addition to signing actions, Lit also provides encryption and decryption capabilities for messages and content. By defining specific decryption conditions for the encrypted content, you can ensure that only qualified parties are allowed to view the decrypted content.
Integrating custom Telegram Authentication to a PKP adds the Telegram user as a party who can prompt the PKP to perform an on-chain action.
For example, a PKP could be used as a Web3 wallet for a user trading bot. A developer could define a Lit Action where the PKP performs an on-chain action once a certain condition is met. The user could define the condition parameters, and the developer could create a web server to check the condition at predetermined intervals. For example:
- When Ether's price exceeds $2,500, the bot could:
- a) Send a message to a Telegram chat, notifying the user to consider buying more Ether.
- b) Propose a potential transaction for user approval.
- Alternatively, once the condition is met, the bot could:
- a) Automatically execute the transaction.
- b) Send a message informing the user that the transaction has been completed.
- c) Provide an update on the PKP's current balance.
Of course, a trading bot is only one of many possible implementations and is by no means the only option.
Implementing Lit
The complete code base for implementing Lit within a Telegram Mini App can be found here. This example shows how to mint a PKP and get session signatures within the Mini App. Next we'll explore some key concepts in the code
Verification of User Data
The initData
value is a string of raw data transferred from the Telegram window.Telegram.WebApp
object to the Mini App. Using this data and the token of our Telegram bot where the Mini App is deployed, we can recreate the hash of this data to compare it with the one provided by Telegram to ensure valid user data. This same process is used within the Lit Action that verifies the user's access to the PKP.
export async function verifyInitData(
telegramInitData: string,
botToken: string
) {
const urlParams: URLSearchParams = new URLSearchParams(telegramInitData);
const hash = urlParams.get("hash");
urlParams.delete("hash");
urlParams.sort();
let dataCheckString = "";
for (const [key, value] of urlParams.entries()) {
dataCheckString += `${key}=${value}\n`;
}
dataCheckString = dataCheckString.slice(0, -1);
const encoder = new TextEncoder();
const secretKey = await window.crypto.subtle.importKey(
"raw",
encoder.encode("WebAppData"),
{ name: "HMAC", hash: "SHA-256" },
false,
["sign"]
);
const botTokenKey = await window.crypto.subtle.sign(
"HMAC",
secretKey,
encoder.encode(botToken)
);
const calculatedHash = await window.crypto.subtle.sign(
"HMAC",
await window.crypto.subtle.importKey(
"raw",
botTokenKey,
{ name: "HMAC", hash: "SHA-256" },
false,
["sign"]
),
encoder.encode(dataCheckString)
);
const calculatedHashHex = Array.from(new Uint8Array(calculatedHash))
.map((b) => b.toString(16).padStart(2, "0"))
.join("");
const isVerified = hash === calculatedHashHex;
return isVerified;
}
Generating Session Signatures
After minting our PKP and adding the Lit Action as a valid authentication method to access the PKP, the example then generates session signatures for the user.
const sessionSignatures = await litNodeClient.getPkpSessionSigs({
pkpPublicKey: pkp.publicKey,
capabilityAuthSigs: [capacityDelegationAuthSig],
litActionCode: Buffer.from(litActionCode).toString("base64"),
jsParams: {
telegramUserData: telegramUser,
telegramBotSecret: import.meta.env.VITE_TELEGRAM_BOT_SECRET,
pkpTokenId: pkp.tokenId,
},
resourceAbilityRequests: [
{
resource: new LitPKPResource("*"),
ability: LitAbility.PKPSigning,
},
{
resource: new LitActionResource("*"),
ability: LitAbility.LitActionExecution,
},
],
expiration: new Date(Date.now() + 1000 * 60 * 10).toISOString(), // 10 minutes
});
This function, taken from the code example, has the following parameters:
pkpPublicKey
: The public key of the PKP that we want to use to sign data.capacityDelegationAuthSig
: An authentication signature used to pay for usage of the Lit network. More information here.litActionCode
: Our custom Telegram authentication within a Lit Action. The complete Lit Action can be found here.jsParams
: A JSON object of the parameters that will be passed to the Lit Action. In this case, we are passing the user's Telegram data, the bot's token, and the PKP's token ID.resourceAbilityRequests
: An array of objects that specify the resources and abilities that the user is requesting. In this case, the ability to sign data with the PKP and execute the Lit Action.
expiration
: The expiration date of the session signatures.
Code Base Example Video
Next Steps
By the end of this guide you should have learned how to successfully integrate Lit into a Telegram Mini App.
We're excited to see the potential of Lit in building chat bots, user wallets, games, and more!
For further learning, explore the Lit Protocol Documentation, Telegram Mini Apps Development Guide, and resources on PKPs and advanced usage of Lit Actions.