Lit Hackathon Resources Spring '23
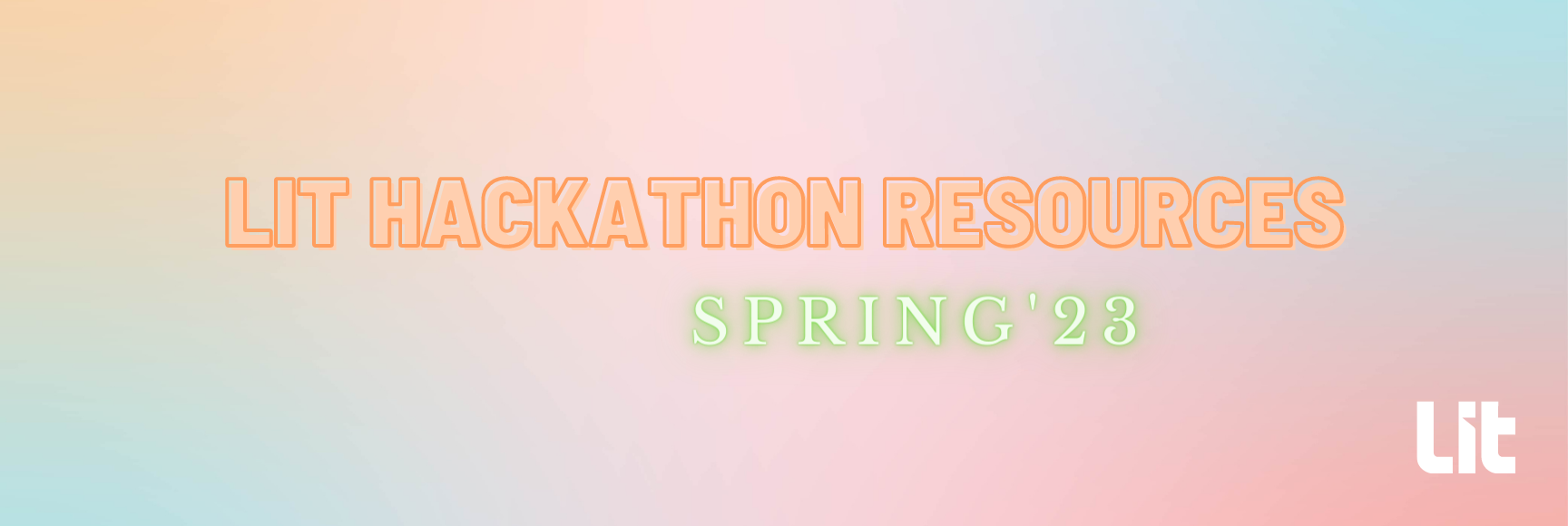
This is a resource guide for developers interested in building with Lit in upcoming hackathons. For the month of April, you’ll be able to find the Lit team at Aragon’s DAO Hackathon and ETHGlobal Tokyo.
To get started, we’ll go over Programmable Key Pairs (PKPs) and Lit Actions. PKPs are ECDSA key-pairs held in distributed custody by the Lit network, and they can be programmed to sign based on application logic specified in a Lit Action. Lit Actions are JavaScript functions that execute across Lit's threshold cryptography network. They are immutable and blockchain agnostic, making them versatile for utilizing on-chain and off-chain data in their computation. Together they enable programmatic signing.
The guide features integral resources of how to interface with the Lit network through the Lit JS SDK. Additionally, developers can use the GetLit CLI Tool to streamline the Lit Actions development process by utilizing a build tool to create and test your functions.
Other resources include an Event Listener Example - Portfolio Rebalancer with Uniswap, which demonstrates how Lit's programmatic signing technology can create an automated portfolio rebalancer that is triggered by a defined set of criteria. Developers can also check out the lightweight PKP oAuth repo, which provides a simple codebase of how to create a Lit-powered MPC wallet solely through Google oAuth.
Wrap up the guide, there will be some code examples from projects in the ecosystem that have integrated Lit. We hope you have fun learning and building!
Introduction to Lit Actions and Programmable Key Pairs (PKPs)
Programmable Key Pairs (PKPs)
A PKP is an ECDSA key-pair that is held in distributed custody by the Lit network. PKPs can be programmed to automatically sign based on application logic, which is specified in a JavaScript program called a Lit Action.
Each PKP is an ECDSA key-pair generated collectively by the Lit nodes through a process called Distributed Key Generation (DKG). As a network, this allows Lit to generate a new keypair where nobody knows the whole private key. Instead, each node only holds a share of the key. These signature shares must be combined above the threshold ( 2/3 of the nodes) to produce the complete signature signed by the PKP.
It’s important to note: only those with authorized access have the ability to request a signature or allow some signing logic to be run. Authorized access refers to the particular "authentication method" used to generate the PKP in the first place, such as a wallet signature or oAuth token.
Lit Actions
Lit Actions are the application logic that a user can ‘add’ to a PKP, this is referred to as programmatic signing.
Specifically, Lit Actions are JavaScript functions that are executed across Lit’s threshold cryptography network. They are JavaScript smart contracts that, when combined with PKPs, can be programmed to sign transactions and other arbitrary data.
Lit Actions are immutable, blockchain agnostic, and can make HTTP requests. This means that they can utilize on-chain and off-chain data in their computation. You can write some code and ask the Lit nodes to execute it, for example asking the network to calculate the difference between two random integers, x and y.
The result of this function can be used to pass some functionality, like signing or provisioning decryption abilities to a user (such as if the difference between x and y is greater than 10, sign the result).
How do PKPs and Lit Actions work together?
A user can create a new PKP and grant a Lit Action the right to sign using it. This means the distributed key has the ability to sign and decrypt arbitrary data based on pre-defined logic and conditions.
You can use the PKPPermissions contract to add additional permitted auth methods and addresses to your PKP. Note that any permitted party will be able to execute transactions and authorize the PKP to sign for Lit Actions.
Resources
JS SDK V2: TypeScript
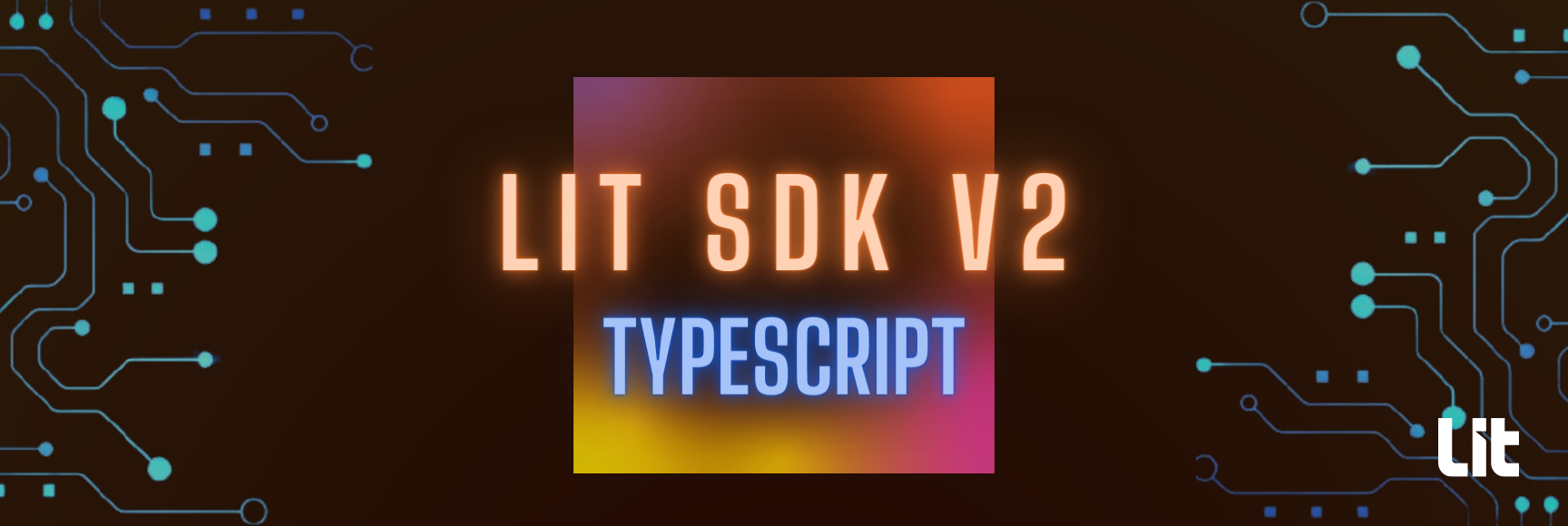
The Lit JS/TS SDK is a powerful tool that empowers developers to seamlessly integrate Lit functionality into their applications. With this SDK, developers can enjoy the full range of features offered by Lit without having to worry about creating their own decentralized threshold cryptography network. Get started today!
How to use the SDK within your project
Importing the SDK:
import * as LitJsSdk from '@lit-protocol/lit-node-client';
Importing a particular module instead of the whole bundled package.
For example, if you only want to get the authSig from the browser, you can use this:
import { checkAndSignAuthMessage } from '@lit-protocol/auth-browser';
More modules can be found in the JS SDK docs.
Core Functionality using the Lit TS SDK:
Access Control
You can use Lit to encrypt and store any static content. This could be a file, a string, or anything that won't change. You need to store the content and metadata yourself (on IPFS, Arweave, or even a centralized storage solution), and Lit will store who is allowed to decrypt it and enforce this (aka key management).
Lit Actions (we’ll cover in the next section)
Quick Start with Lit Actions and PKPs
Get started with Lit Actions and Programmable Key Pairs (PKPs).
Here's a brief rundown to help you get started quickly and easily with your first Lit Actions and PKP:
- Obtain a PKP from the PKP Explorer. PKPs can be programmed to automatically sign based on application logic, which is specified in a Lit Action. You will use the public key of your PKP to sign the output of a Lit Action.
- Write a simple Lit Action that requests a signature from the Lit nodes on the string "Hello World" using the PKP public key obtained from the previous step. Check out the Hello World example for guidance.
const go = async () => {
// this is the string "Hello World" for testing
const toSign = [72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100];
// this requests a signature share from the Lit Node
// the signature share will be automatically returned in the HTTP response from the node
const sigShare = await Lit.Actions.signEcdsa({
toSign,
publicKey:
"0x0404e12210c57f81617918a5b783e51b6133790eb28a79f141df22519fb97977d2a681cc047f9f1a9b533df480eb2d816fb36606bd7c716e71a179efd53d2a55d1",
sigName: "sig1",
});
};
go();
3. Create a function that calls the Lit nodes and asks to sign the “Hello World” array.
To call the Lit nodes to execute the Lit Action, you'll need to use call executeJS
which execute JS on the nodes, combines and return any resulting signatures. Read the API docs here.
const runLitAction = async () => {
// you need an AuthSig to auth with the nodes
// this will get it from MetaMask or any browser wallet
const authSig = await LitJsSdk.checkAndSignAuthMessage({ chain: "ethereum" });
const litNodeClient = new LitJsSdk.LitNodeClient({ litNetwork: "serrano" });
await litNodeClient.connect();
const signatures = await litNodeClient.executeJs({
code: litActionCode,
authSig,
// all jsParams can be used anywhere in your litActionCode
jsParams: {
// this is the string "Hello World" for testing
toSign: [72, 101, 108, 108, 111, 32, 87, 111, 114, 108, 100],
publicKey:
"{YOUR PKP PUBLIC KEY}",
sigName: "sig1",
},
});
console.log("signatures: ", signatures);
};
runLitAction();
4. You’ve written your first Lit Actions function! Run it and the PKP will sign!
5. Explore these additional examples for more ideas and inspiration:
- Conditional Signing: This example demonstrates how to use conditional logic to sign a message only if certain conditions are met.
- Using Fetch: This example shows how to use the fetch API to make HTTP requests within a Lit Action.
GetLit CLI Tool
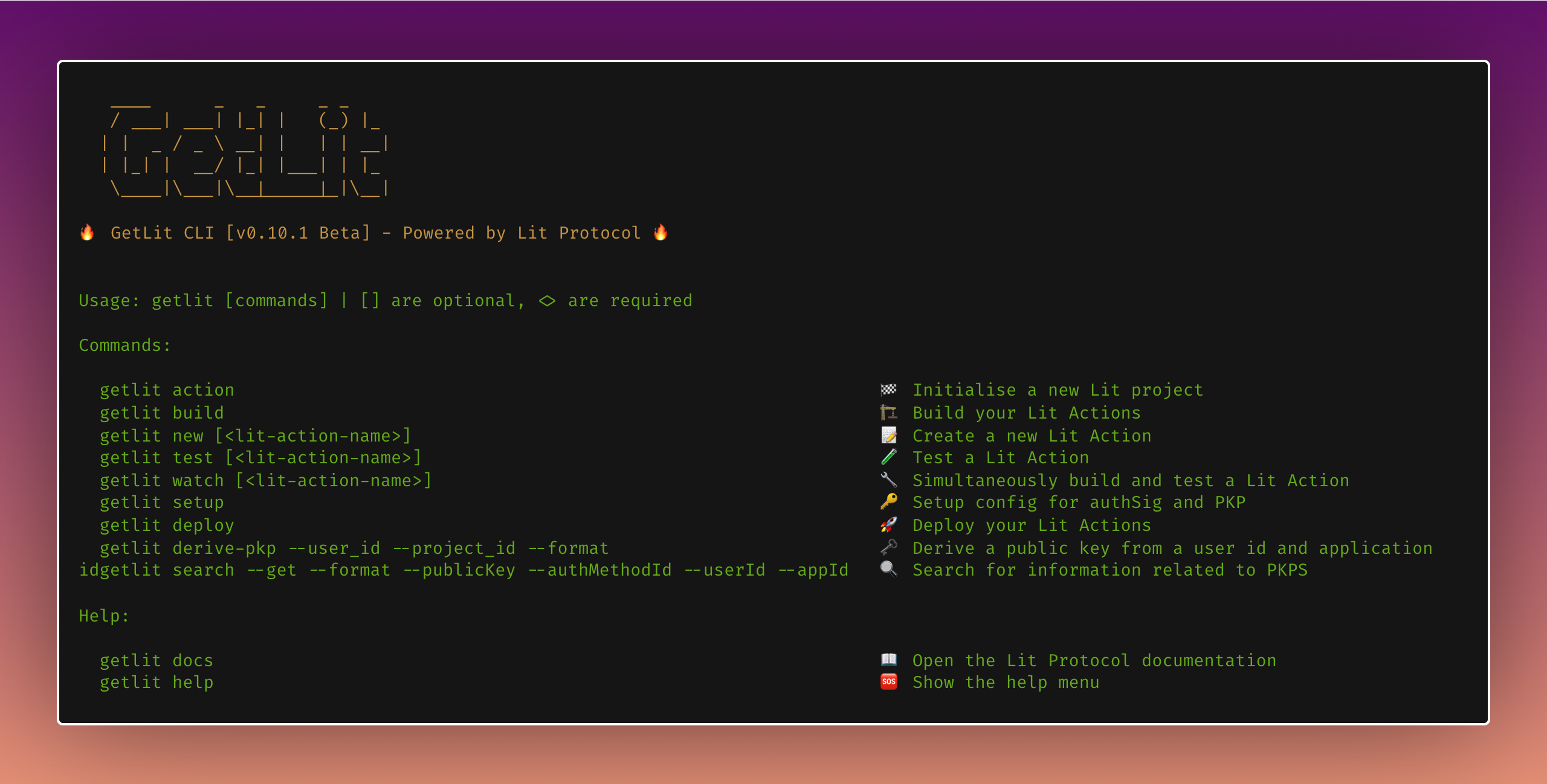
GetLit CLI | Lit Protocol Developer Docs
Purpose:
The GetLit CLI is a command-line tool designed to help developers manage their Lit Actions functions. The CLI provides a set of commands to create, build, test, and configure Lit Actions.
The aim of this project is to develop and implement Lit Actions that can be easily tested and built upon. By making it easier to test and build, we hope to reduce the time and effort required to create high-quality applications with Lit. This will allow developers to focus on more important aspects of their work, such as designing intuitive user interfaces and optimizing performance.
Getting started
npm install -g getlit
// or
yarn add global getlit
Some helpful commands, to check out the full list of commands go here.
Command | Usage | Description |
---|---|---|
getlit init | here | getlit init |
getlit build | getlit build | 🏗 Build your Lit Actions |
getlit new | action | getlit new [ |
getlit test | getlit test [ |
🧪 Test a Lit Action |
To use the GetLit CLI, simply run the desired command followed by any required or optional arguments. The CLI will execute the associated function and display the output accordingly.
Event Listener Example - Portfolio Rebalancer with Uniswap
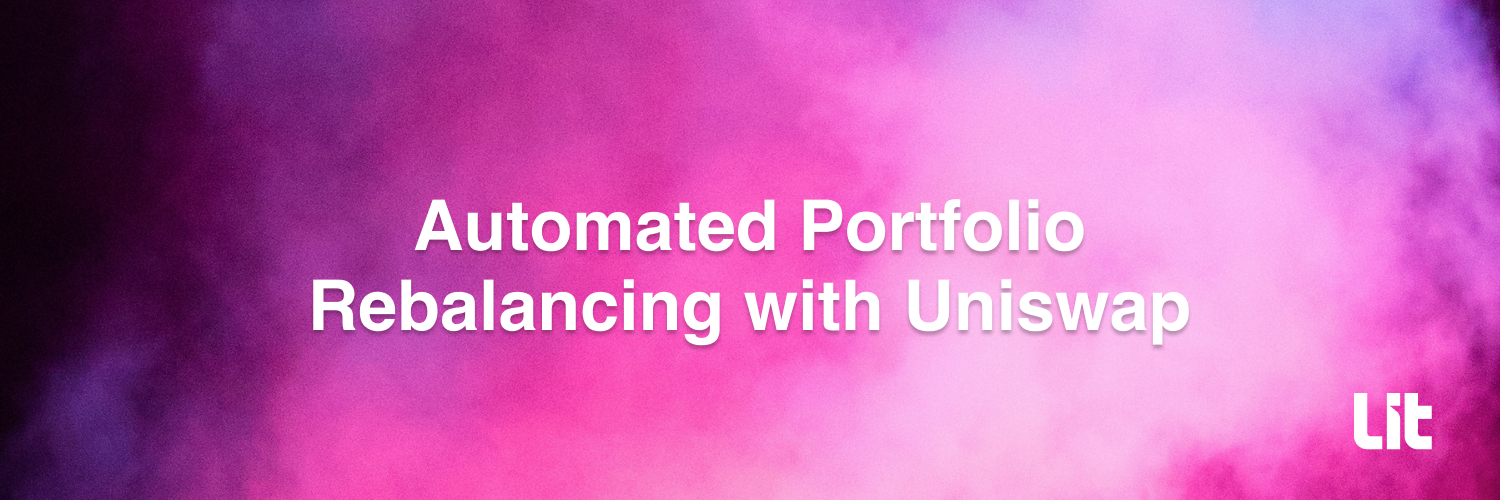
Purpose:
The purpose of this section is to highlight how developers can leverage Lit's programmatic signing technology to create an automated portfolio rebalancer that is triggered by a defined set of criteria. This guide aims to showcase how an event listener can kick off programmed signing and trading with Uniswap.
Uniswap is a popular decentralized exchange protocol that enables users to trade without intermediaries. By leveraging Lit's programmatic signing technology, developers can create a streamlined and automated process for trading on Uniswap based on pre-defined criteria.
Lit MPC Wallet Created with Google oAuth
This project lets you start using Ethereum with just a Google Account. It's a simple example of how to use the Lit Protocol Oauth service to authenticate users and then use their Ethereum address.
This project specifically:
- Uses Google oAuth to auth the user
- Mints a PKP token for the user, with their Google account as a valid auth method
- Uses the PKP token to get an Ethereum address for the user
- Generates a local session key for the user and stores it in LocalStorage
- Uses the Lit Protocol's PKP Session Signing service to sign that session key with their PKP
- Uses the local session key to sign a request to encrypt and decrypt a string that only the user can decrypt.
The purpose of this codebase is to provide a simple codebase of how to create a Lit powered MPC wallet with Google oAuth. Check out the live demo.
Connect Your Lit-Powered Cloud Wallet to the Decentralized Web
If you're looking to connect your Lit PKP to dApps via WalletConnect, you're in luck! With the live demo available here, it's now easier than ever to get started.
For those who want to learn more about the core pieces necessary to integrate Lit PKPs and WalletConnect V1 in your app, be sure to check out this comprehensive guide. The guide covers everything from the basics of Lit PKPs to the intricacies of integrating WalletConnect V1.
If you're interested in diving deeper, take a look at the code to gain a better understanding of the technology behind the integration process.
Ecosystem Examples
Open source projects built with Lit to check out
Orbis - Web3 Social Hackathon
The Orbis Web3 Social hackathon was an exciting event that brought together developers from all over the world to collaborate and create innovative solutions within the web3 social space.
One of the projects featured from the hackathon is the Ceramic multi users project. This project allows multiple wallets and authorized parties to write to a Ceramic stream, something that is not currently supported by Ceramic. Check out the GitHub repo.
Another project showcased at the Orbis hackathon is IFTTT for Orbis, which is a tool that enables users to automate Orbis actions via Lit PKPs and Lit Actions. The actions are triggered by numerous web2 and web3 services such as automating a post on Orbis through setting a calendar event. Source code and more information on the project can be found in the GitHub repo.
Finally, the winner of the Lit prize, Magic Wallet! The project utilizes a PKP to access and execute all the functions of the Orbis Protocol, including sending transactions through a message monitoring system and connecting to other dApps like Uniswap to trade assets. Check out the project on GitHub.
ETHDenver
Intuition - Slang - Finalist
Slang is a powerful tool for developers that allows them to work with decentralized databases with ease. Built on top of ComposeDB and Prisma, the goal of the project is to simplify interacting with decentralized databases. In order to do that, the project utilizes Programmable Key Pairs (PKPs), and Lit Actions to simplify decentralized database interactions. Check out the code here and use it in your project!
Yacht Labs - Cross Chain Bridging with Yacht x Lit
Transferring assets across chains should be trustless, verifiable, and instantaneous. Given that we can verify data on-chain and use a Lit key pair to sign transactions, we can open up a world of possibilities when it comes to cross chain interactions.
The Yacht SDK generates a PKP and writes some code to check the balance of its public address, which allows the development of an escrow service where two parties both send assets to the PKP address. Then the Lit network can generate two transactions sending the respective tokens to each counterparty. This becomes a trustless, decentralized and near instantaneous swap of assets between chains. The moment that the PKP verifies that it has the required balance of the two tokens on both chains, the swap can be completed. No more waiting for optimistic rollups or hoping your exchange’s KYC goes through. Just decide on a price, send your assets to the PKP, and you’re good to go.
Cross Chain Access Control Lists with WeaveDB and Lit
Lit enables WeaveDB to build access control logic using states from multiple blockchains combined, and encrypt data so only certain addresses that satisfy the access control rules can decrypt it.
For example, you can build a messaging dApp where you can send encrypted messages to only specified NFT holders. And only the holders of specified tokenIDs will be able to decrypt your messages. Learn more through WeaveDB's docs.
Fin
We hope this resource guide has been useful for developers interested in building with Lit. As always, the Lit team is available to help through Discord, Lenster or Twitter. Happy hacking!
Don't forget to keep an eye out for upcoming hackathons where you can put your skills to the test and create innovative projects using Lit's programmatic signing technology. Lit is running The Ignite Metaprize - one project started at a hackathon - will win $20,000 at the end of the year (read more for guidelines and rules). We can't wait to see what you build!